How Developers Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
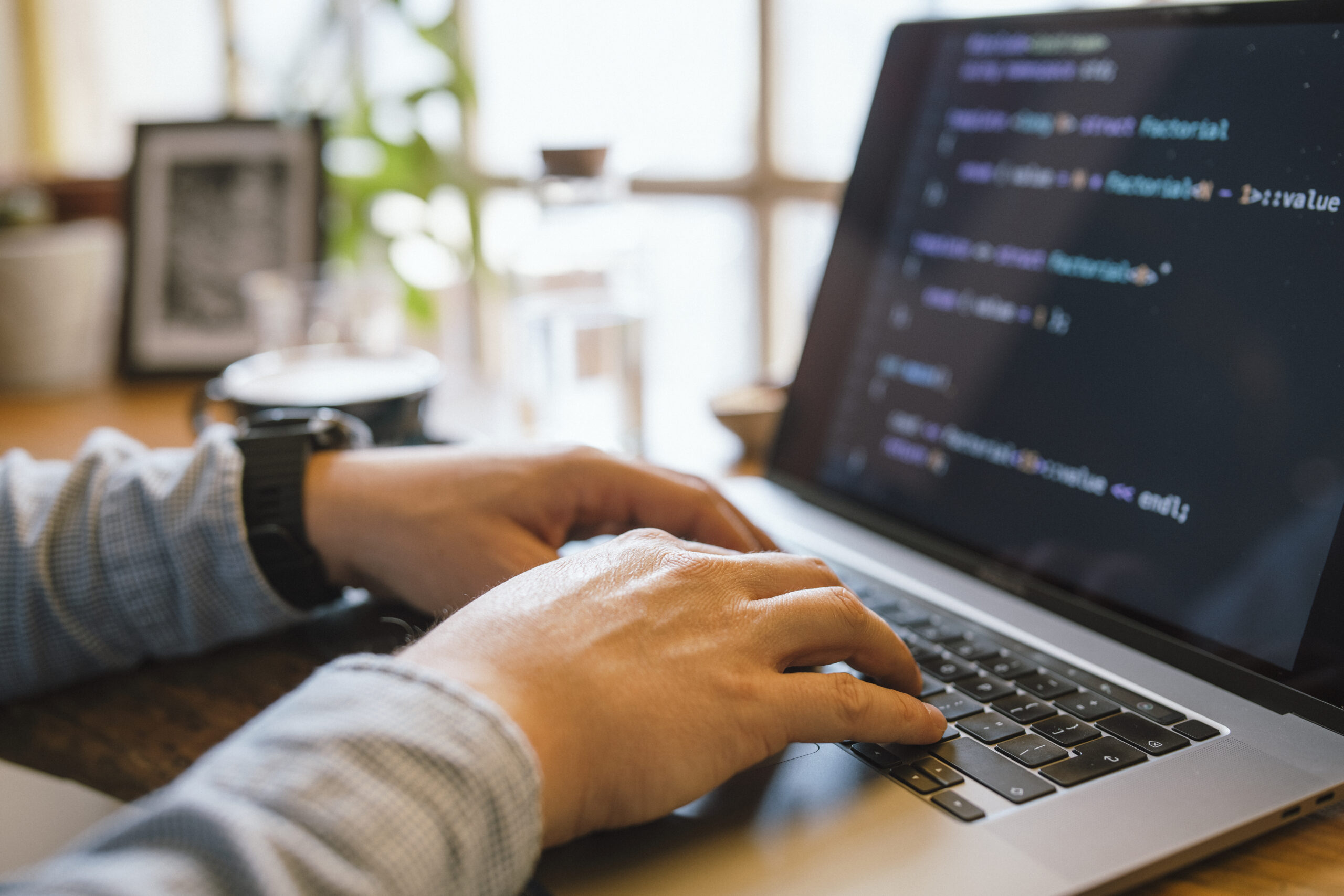
Debugging is Among the most vital — nonetheless often disregarded — capabilities in a very developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Understanding to Consider methodically to resolve troubles successfully. Irrespective of whether you are a beginner or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially help your efficiency. Here i will discuss quite a few procedures that can help builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways developers can elevate their debugging skills is by mastering the applications they use on a daily basis. When composing code is a single Section of development, recognizing tips on how to communicate with it efficiently throughout execution is Similarly important. Modern-day growth environments come Geared up with strong debugging capabilities — but numerous builders only scratch the surface area of what these tools can perform.
Get, for instance, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and in some cases modify code on the fly. When applied appropriately, they Permit you to notice specifically how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, for instance Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, watch actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can change discouraging UI problems into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Regulate units like Git to know code background, uncover the precise minute bugs were being launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about producing an personal expertise in your enhancement environment so that when problems arise, you’re not missing in the dead of night. The higher you recognize your instruments, the greater time it is possible to commit resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
One of the most critical — and infrequently forgotten — techniques in powerful debugging is reproducing the challenge. Just before leaping to the code or creating guesses, developers have to have to make a steady atmosphere or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a activity of probability, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as is possible. Request questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the exact problems beneath which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the trouble in your local setting. This could indicate inputting the same knowledge, simulating similar person interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or condition transitions associated. These tests not merely assistance expose the trouble and also prevent regressions Down the road.
Occasionally, The problem can be environment-certain — it would materialize only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t simply a step — it’s a attitude. It calls for patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be now midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and connect extra Evidently with all your workforce or buyers. It turns an summary criticism into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Error messages tend to be the most respected clues a developer has when some thing goes wrong. Rather than looking at them as discouraging interruptions, builders really should understand to deal with error messages as immediate communications through the program. They frequently show you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Start off by reading through the concept cautiously As well as in entire. Numerous builders, particularly when under time force, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the error down into pieces. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it point to a certain file and line number? What module or functionality induced it? These questions can information your investigation and point you towards the liable code.
It’s also beneficial to be familiar with the terminology with the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently comply with predictable styles, and Understanding to acknowledge these can drastically increase your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to look at the context by which the error transpired. Look at associated log entries, input values, and up to date modifications while in the codebase.
Don’t forget compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Correctly
Logging is Among the most impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase through the code line by line.
A good logging strategy starts off with understanding what to log and at what degree. Typical logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in-depth diagnostic information and facts all through progress, Details for standard activities (like productive begin-ups), Alert for potential challenges that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your process. Give attention to important situations, point out alterations, input/output values, and important selection points as part of your code.
Format your log messages Evidently and constantly. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in generation environments where stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a perfectly-believed-out logging technique, you can decrease the time it will require to identify problems, achieve further visibility into your applications, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a specialized undertaking—it is a form of investigation. To proficiently detect and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude allows break down complicated concerns into manageable areas and follow clues logically to uncover the root result in.
Get started by accumulating evidence. Look at the signs of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, obtain just as much pertinent details as it is possible to devoid of leaping to conclusions. Use logs, take a look at situations, and consumer stories to piece jointly a transparent photograph of what’s occurring.
Following, kind hypotheses. Question by yourself: What could possibly be leading to this behavior? Have any changes recently been created towards the codebase? Has this issue happened in advance of beneath identical instances? The purpose is always to narrow down alternatives and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue in a managed surroundings. If you suspect a selected operate or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and let the final results lead you nearer to the truth.
Pay shut consideration to little facts. Bugs usually hide while in the least predicted locations—just like a missing semicolon, an off-by-a person error, or simply a race issue. Be thorough and affected individual, resisting the urge to patch the issue devoid of completely being familiar with it. Short-term fixes may perhaps cover the real difficulty, just for it to resurface later.
And finally, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can preserve time for long run problems and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be more effective at uncovering hidden concerns in advanced systems.
Create Exams
Producing checks is among the most effective methods to transform your debugging skills and General advancement effectiveness. Assessments don't just help catch bugs early but additionally serve as a safety Internet that offers you assurance when earning changes for your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint specifically in which and when a challenge takes place.
Begin with device exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether a particular piece of logic is working as envisioned. Any time a take a look at fails, you promptly know the place to seem, substantially lowering the time used debugging. Device checks are In particular valuable for catching regression bugs—concerns that reappear following Formerly becoming fixed.
Future, combine integration exams and end-to-conclusion checks into your workflow. These support make certain that numerous aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with multiple parts or providers interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with By natural means potential customers to better code framework and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug can be a strong starting point. After the take a look at fails consistently, you'll be able to deal with fixing the bug and look at your exam move when The difficulty is fixed. This method makes sure that a similar bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a aggravating guessing video game right into a structured and predictable procedure—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to become immersed in the situation—gazing your monitor for hours, trying Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, minimize stress, and sometimes see The problem from a new viewpoint.
When you are way too near to the code for way too extensive, cognitive exhaustion sets in. You would possibly get started overlooking noticeable glitches or misreading code you wrote just hrs previously. On this state, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avoid burnout, Particularly during extended debugging periods. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You would possibly abruptly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
When you’re stuck, a fantastic rule of thumb will be to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move all over, stretch, or do something unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is just not an indication of weakness—it’s a wise method. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Just about every bug you encounter is more than just A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something beneficial in case you make the effort to reflect and evaluate what went Mistaken.
Start out by inquiring yourself a couple of crucial inquiries when the bug is solved: website What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The answers frequently reveal blind spots in your workflow or understanding and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially potent. Whether it’s via a Slack information, a short create-up, or A fast expertise-sharing session, assisting others stay away from the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Additional importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as critical areas of your development journey. In spite of everything, a few of the best builders are not those who generate best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So up coming time you squash a bug, have a moment to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.